Using LUA in Demoniak3D
LUA/HYP HOST -API initiation
By Jérôme 'JeGX' GUINOT - The oZone3D Team
jegx [at] ozone3d [dot] net
Initial draft: October 10, 2005
Update: October 21, 2005
Translated from french by Samir Fitouri - soundcheck [at] ozone3d [dot] net
Prerequisite
1 - Introduction

The
Host-API (API for Application Programming Interface) Demoniak3D/LUA is the whole of the functions which allow a script
written in the LUA scripting language to interact with
Demoniak3D.
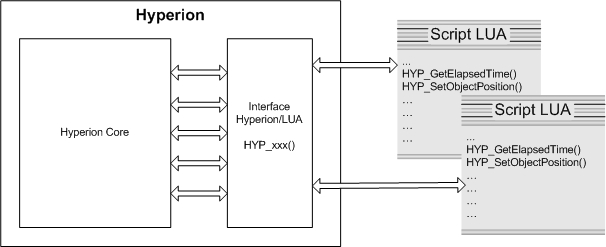
Fig. 1 - Host-API LUA.Demoniak3D uses the
script node (XML element) to integrate some LUA scripting code in the 3d scenes. LUA code can be written either
in LUA files (* lua), or directly within the
raw_data element of the script node.
The working of Demoniak3D can be modelled by the following diagram:
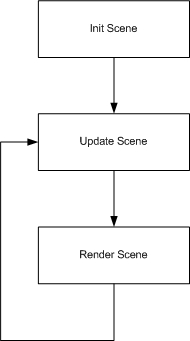
Fig. 2 - Global operating of Demoniak3DLUA scripts intervene in the 2 following stages:
- the scene initialization
- the scene update
The
initialization stage is performed only once, just after loading all the medias that are necessary to the 3d scene
(models, textures...). The
update stage, like the rendering stage, is continuously (infinitely) performed several tens of time
per second. The model of figure 2 is not specific to Demoniak3D, it is that used by the majority of the video games. A simple operating
model but of a formidable effectiveness!
There are two types of LUA scripts in Demoniak3D, which rise from the model of figure 2:
- initialization scripts: these scripts are runt ONLY ONCE in the scene initialization stage. These scripts are generally used to
initialize the data (global variables). They have an operating mode (run_mode) called EXECUTE_ONCE.
- update scripts: these scripts are run before each scene rendering. These scripts contain all the logic of your demonstration, the
updates of the 3d objects position, physics handling, AI (Artificial Intelligence), audios tracks. The run_mode of these scripts is EXECUTE_EACH_FRAME.
From this information, one can describe the evolution of a 3d scene:
- XML and LUA initialization scripts describe the state of the scene at time t zero. All these scripts define the initial
conditions of the scene.
- update scripts describe the state of the scene at a time t higher than zero. LUA updates scripts are not necessary to all the scenes.
For simple scenes, the initial conditions are sufficient to create a dynamic scene (see for example the auto_spin attribute of some
objects such as the 3d models).
2 - Libraries

Host-API LUA is mainly made up of libraries. These libraries are distributed according to the functionalities which are
available in Demoniak3D. Here are the principal ones:
- management of the properties common to all 3d objects: HYP_Object library.
- management of 3d meshes and models: HYP_Model and HYP_Mesh libraries.
- management of cameras: HYP_Camera library.
- management of lights: HYP_Light library.
- management of materials: HYP_Material library.
- management of textures: HYP_Texture library.
- management of user input: HYP_Input library.
- management of audio tracks: HYP_Sound library.
- ...
For a complete and detailed description, please refer to the Reference Guide provided with Demoniak3D.
The use of a library is easy. The following example shows how to modify the position of an object (called mySphere) by using
the HYP_Objetc library:
HYP_Object.SetPosition( "mySphere", 12.0, 50.0, -100.0 );
3 - Example

Now that we have the bases of LUA and the Host-API, let us analyse a concrete example. We will use for that the
code sample 41.
This example shows how to generate a matrix of spheres.
The matrix needing to be created only once, its creation will be done in a LUA script whose run_mode is set to EXECUTE_ONCE.
The second LUA script will make it possible to drive an omni type dynamic light around the matrix. The position of the light having
to be updated with each frame, this script will have a run_mode set to EXECUTE_EACH_FRAME.
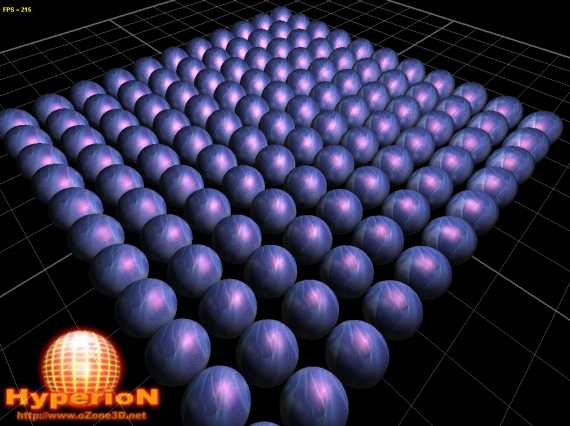
Fig. 3 - code sample 41The initilalisation script is the following one:
<script name="init_scene" run_mode="EXECUTE_ONCE" >
<raw_data>
<![CDATA[
clone_id = -1;
for j=0,10 do
z = -100.0 + j*20.0;
for i=0,10 do
clone_id = HYP_Object.Clone("master_sphere");
x = -100.0 + i*20.0;
HYP_Object.SetRenderState( clone_id, 1);
HYP_Object.SetLightingState( clone_id, 1 );
HYP_Object.SetTexturingState( clone_id, 1 );
HYP_Object.SetPosition( clone_id, x, 0.0, z);
HYP_Object.RemoveSeam( clone_id );
HYP_Object.SetVBOState( clone_id, 1 );
end
end
]]>
</raw_data>
</script>
The goal of this script is to clone 100 times a mesh sphere (called master_sphere and declared in the main XML script) and to
position each clone in order to form the matrix of figure 3. This script is directly written within the XML code by using
the raw_data element.
In LUA coding, a 3d entity can be identified either by a string character (in general the name given in the XML script with the name
attribute) or by an integer number. Most of the functions of the various libraries host-api accept these two ways to identify
a 3D entity. In the previous code, the HYP_Object.Clone() function returns a numerical identifyer of the new cloned object which will be
used in the next functions.
The light source movement is managed by the following script:
<script name="update_scene" run_mode="EXECUTE_EACH_FRAME" >
<raw_data>
<![CDATA[
elapsed_time = HYP_GetElapsedTime()*0.001;
xpos = 80.0 * math.cos(elapsed_time*0.4);
ypos = 90.0 * math.cos(-elapsed_time*0.4);
zpos = 120.0 * math.sin(elapsed_time*0.4);
HYP_Object.SetPosition( "light_01", xpos, ypos, zpos );
]]>
</raw_data>
</script>